Visual Basic 2008 Code Examples Pdf
It’s time to write an application that puts together the most important topics discussed in this section. The MultipleForms example consists of a main form, an auxiliary form, and a dialog box. All three components of the application’s interface are shown in Figure 5.12. The buttons on the main form display both the auxiliary form and the dialog box.
Visual Basic Projects, Visual Basic Examples, Visual Basic Source Code Search form Visual Basic is a third-generation event-driven programming language and integrated development environment (IDE) from Microsoft for its COM programming model first released in 1991. Visual Basic 2008 is Microsoft’s latest incarnation of the enormously popular Visual Basic language, and it’s fundamentally different from the versions that came before it. Visual Basic 2008 is now more powerful and more capable than ever before, and its features and functionality are on par with “higher-level” languages such as C.
Let’s review the various operations we want to perform — they’re typical for many situations, not for only this application. At first, we must be able to invoke both the auxiliary form and the dialog box from within the main form; the Show Auxiliary Form and Show Dialog Box buttons do this. The main form contains a variable declaration: strProperty. This variable is, in effect, a property of the main form and is declared as public with the following statement:
The main form calls the auxiliary form’s Show method to display it in a modeless manner. The auxiliary form button named Read Shared Variable In Main Form reads the strProperty variable of the main form with the following statement:
Using the same notation, you can set this variable from within the auxiliary form. The following event handler prompts the user for a new value and assigns it to the shared variable of the main form:
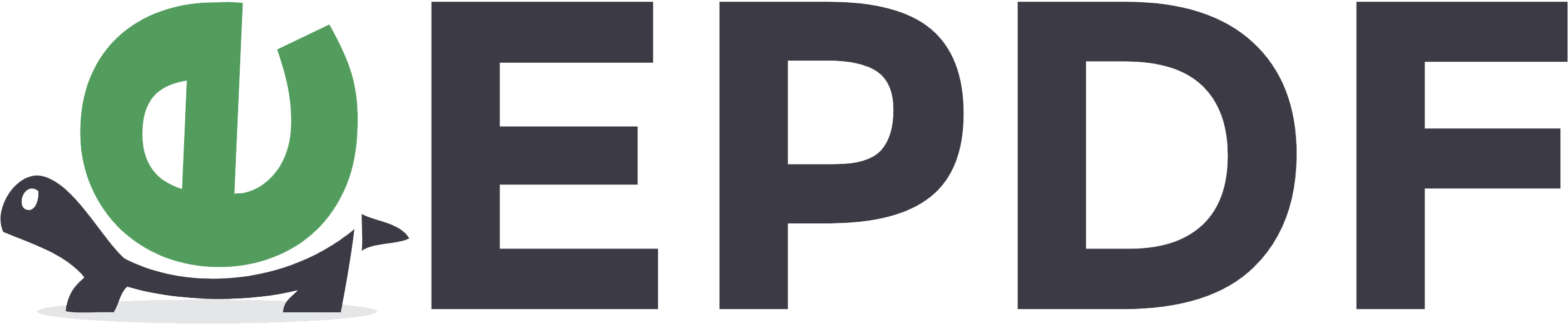
The two forms communicate with each other through public variables. Let’s make this communication a little more elaborate by adding an event. Every time the auxiliary form sets the value of the strProperty variable, it will raise an event to notify the main form. The main form, in turn, will use this event to display the new value of the string on the TextBox control as soon as the code in the auxiliary form changes the value of the variable and before it’s closed.
Figure 5.12 – The MultipleForms Example Interface
To raise an event, you must declare the event’s name in the form’s declaration section. Insert the following statement in the auxiliary form’s declarations section:
Now add a statement that fires the event. To raise an event, we call the RaiseEvent statement, passing the name of the event as an argument. This statement must appear in the Click event handler of the Set Shared Variable In Main Form button, right after setting the value of the shared variable. Listing 5.4 shows the revised event handler.
Listing 5.4: Raising an Event

The event will be raised, but it will go unnoticed if we don’t handle it from within the main form’s code. To handle the event, you must create a variable that represents the auxiliary form with the WithEvents keyword:
The WithEvents keyword tells VB that the variable is capable of raising events. If you expand the drop-down list with the objects in the code editor, you will see the name of the FRM variable, along with the other controls you can program. Select FRM in the list and then expand the list of events for the selected item. In this list, you will see the strPropertyChanged event.
Select it, and the definition of an event handler will appear. Enter these statements in this event’s handler:
Visual Basic 2008 Code Examples Pdf Template
It’s a simple handler, but it’s adequate for demonstrating how to raise and handle custom events on the form level. If you want, you can pass arguments to the event handler by including them in the declaration of the event. To pass the original and the new value through the strPropertyChanged event, use the following declaration:
If you run the application now, you’ll see that the value of the TextBox control in the main form changes as soon as you change the property’s value in the auxiliary form.
Of course, you can update the TextBox control on the main form directly from within the auxiliary form’s code. Use the expression MainForm.TextBox1 to access the control and then manipulate it as usual. Events are used to perform some actions on a form when an action takes place in one of the other forms of the application. The benefit of using events, as opposed to accessing members of another form from within our code, is that the auxiliary form need not know anything about the form that called it. The auxiliary form raises the event, and it’s the other form’s responsibility to handle it.
Let’s see now how the main form interacts with the dialog box. What goes on between a form and a dialog box is not exactly interaction; it’s a more timid type of behavior. The form displays the dialog box and waits until the user closes the dialog box. Then it looks at the value of the DialogResult property to find out whether it should even examine the values passed back by the dialog box. If the user has closed the dialog box with the Cancel (or an equivalent) button, the application ignores the dialog box settings. If the user closed the dialog box with the OK button, the application reads the values and proceeds accordingly.
Before showing the dialog box, the code of the Show Dialog Box button sets the values of certain controls in it. In the course of the application, it usually makes sense to suggest a few values in the dialog box, so that the user can accept the default values by pressing the Enter key. The main form selects a date on the dialog box’s controls and then displays the dialog box with the statements given in Listing 5.5.
Listing 5.5: Displaying a Dialog Box and Reading Its Values
Sample Visual Basic Program Code
To close the dialog box, you can click the OK or Cancel button. Each button sets the DialogResult property to indicate the action that closed the dialog box. The code behind the two buttons is shown in Listing 5.6.
Listing 5.6: Setting the Dialog Box’s DialogResult Property
Because the dialog box is modal, the code in the Show Dialog Box button is suspended at the line that shows the dialog box. As soon as the dialog box is closed, the code in the main form resumes with the statement following the one that called the ShowDialog method of the dialog box. This is the If statement in Listing 5.5 that examines the value of the DialogResult property and acts accordingly.